NVIDIA’s GH200 Grace Hopper Superchip Enhances Generative AI

[ad_1]
In an period the place expertise repeatedly pushes boundaries, Nvidia has as soon as once more left its mark. The corporate has launched the GH200 Grace Hopper Superchip, a complicated AI chip tailor-made to amplify generative AI functions. This newest innovation guarantees to revolutionize AI, providing enhanced efficiency, reminiscence, and capabilities to propel AI to heights.
Additionally Learn: China’s Hidden Marketplace for Highly effective Nvidia AI Chips
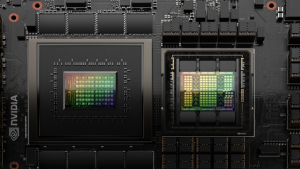
Unveiling the GH200 Grace Hopper: A New Period of AI
Nvidia, a famend chip producer, has launched the world to its cutting-edge GH200 Grace Hopper platform, a next-generation AI chip designed for accelerated computing. This platform will deal with probably the most intricate generative AI workloads, starting from complicated language fashions to recommender methods and complicated vector databases.
Additionally Learn: NVIDIA Builds AI SuperComputer DGX GH200
A Glimpse into Grace Hopper Superchips
On the coronary heart of the GH200 Grace Hopper platform lies the revolutionary Grace Hopper Superchip. This groundbreaking processor incorporates the world’s first HBM3e (Excessive Bandwidth Reminiscence 3e) expertise, providing a mess of configurations to cater to numerous wants. Nvidia’s founder and CEO, Jensen Huang, lauds the platform’s outstanding reminiscence expertise, seamless efficiency aggregation via GPU connections, and an adaptable server design that facilitates information center-wide deployment.
Additionally Learn: SiMa.ai to Carry World’s Most Highly effective AI Chip to India
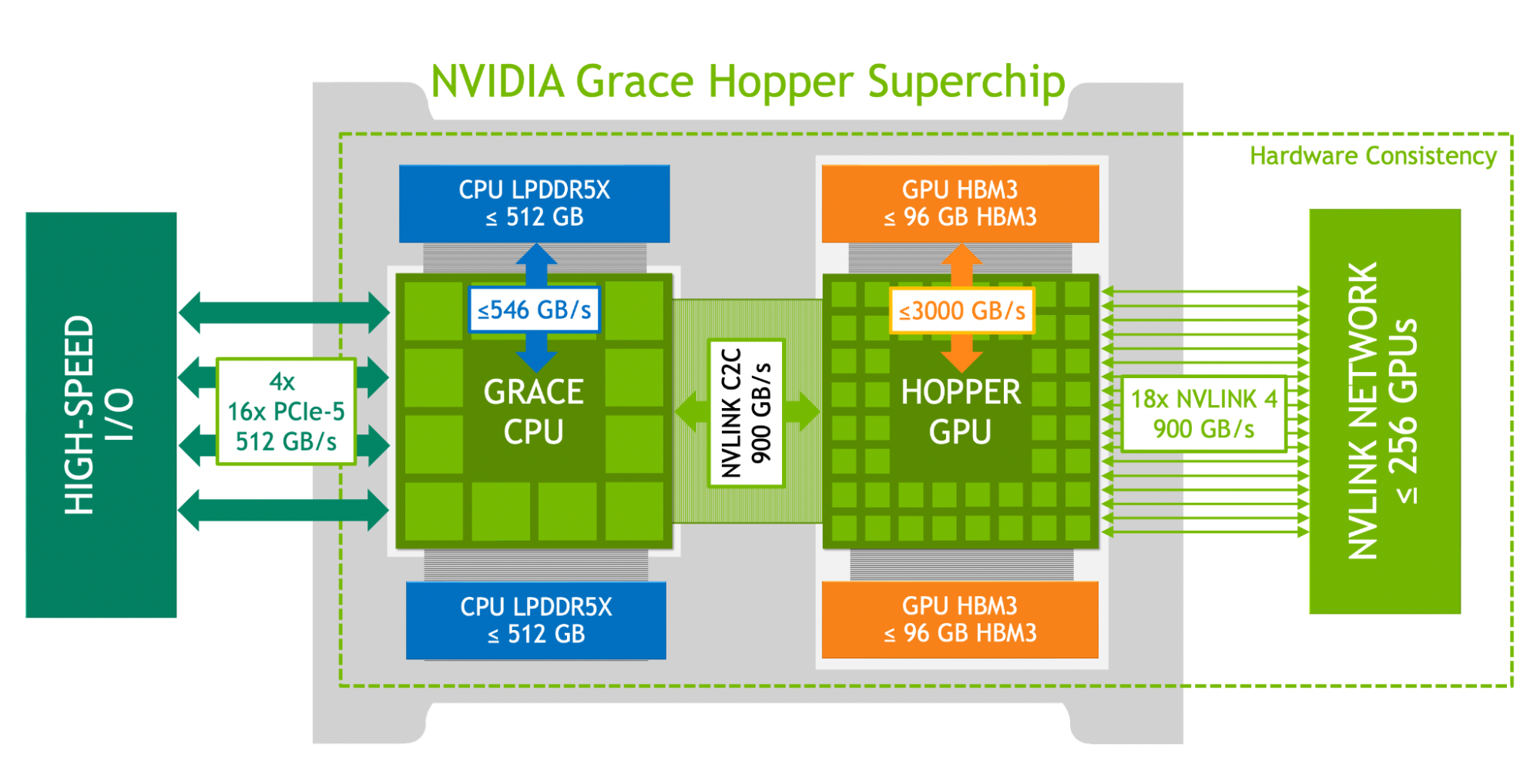
NVIDIA NVLink: Paving the Path for Collaborative Energy
Nvidia has as soon as once more outdone itself by enabling the Grace Hopper Superchip to attach with further Superchips utilizing Nvidia NVLink expertise. This breakthrough connectivity empowers a number of chips to hitch forces, seamlessly deploying colossal generative AI fashions that had been beforehand unimaginable. The high-speed NVLink ensures that the GPU positive aspects full entry to CPU reminiscence, culminating in a formidable 1.2TB of quick reminiscence in dual-chip configurations.
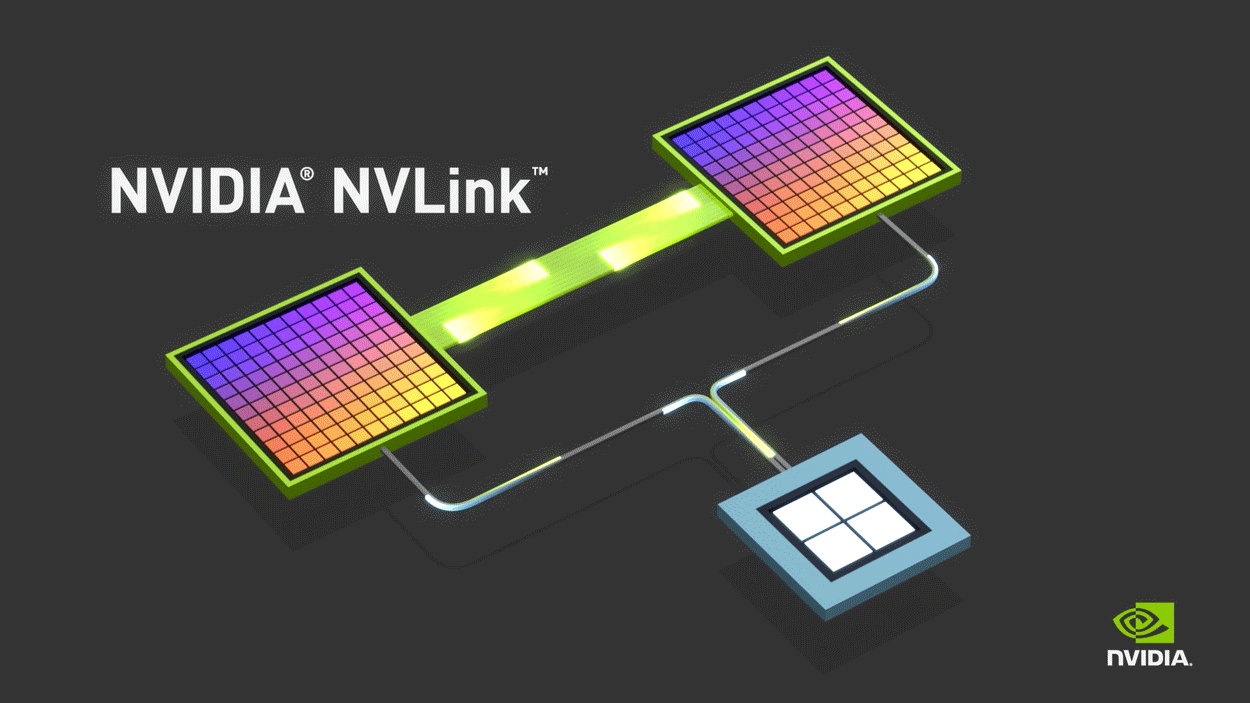
Amplified Efficiency: A Leap in Reminiscence Bandwidth
The introduction of HBM3e reminiscence is a pivotal second in AI development. With a staggering 50% improve in reminiscence bandwidth in comparison with its predecessor, HBM3e delivers an astonishing 10TB/sec mixed bandwidth. This enhance in reminiscence capability empowers the GH200 Grace Hopper platform to deal with fashions 3.5 occasions bigger than its predecessors whereas making certain an unparalleled efficiency enhance.
Additionally Learn: Samsung Embraces AI and Massive Information, Revolutionizes Chipmaking Course of
Empowering Companies: The Street Forward
Main system producers are gearing as much as launch platforms primarily based on the GH200 Grace Hopper within the second quarter of 2024. Nvidia’s unwavering dedication to empowering information facilities and enhancing AI improvement is obvious via this revolutionary product. Because the AI panorama evolves, Nvidia’s {hardware} improvements proceed to reshape the boundaries of risk.
Additionally Learn: Authorities Intervention in Chip Design: A Boon or Bane for India’s Semiconductor Ambitions?
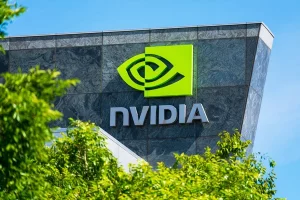
Revolutionizing AI Coaching and Inference
The GH200 Grace Hopper platform’s potential extends to the coaching and fine-tuning massive language fashions. With the flexibility to deal with fashions with a whole lot of billions of parameters, and the prospect of quickly exceeding a trillion parameters, Nvidia’s {hardware} innovation simplifies AI improvement. This development means AI fashions could be educated and referenced utilizing compact networks, eliminating the necessity for in depth supercomputing assets.
Additionally Learn: New AI Mannequin Outshine GPT-3 with Simply 30B Parameters
A Visionary Future for AI
Nvidia’s imaginative and prescient for AI transcends simply {hardware}. As AI turns into extra integral to our lives, Nvidia introduces a spread of AI architectures catering to numerous AI wants. Collaborations with tech giants similar to Microsoft, AWS & Google Cloud showcase Nvidia’s position as an AI {hardware} supplier of alternative. Nvidia’s dedication to AI infrastructure, effectivity, and efficiency is obvious in its Spectrum-X Ethernet platform, which propels information processing and AI workloads within the cloud.
Our Say
By launching the GH200 Grace Hopper platform, Nvidia has as soon as once more confirmed its prowess in driving the AI revolution. Nvidia empowers AI builders and companies to push boundaries and discover new horizons by offering unparalleled reminiscence, efficiency, and connectivity. As Nvidia’s improvements proceed to form the AI panorama, we are able to anticipate an period of unprecedented AI achievements and discoveries.
Associated
[ad_2]